This will check for two 5-digit numbers separated by a comma
^\d{5},\d{5}$
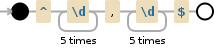
But, you said at least two, so that means it needs to be a little more flexible to accommodate more. If the user enters 12345,12345,12345
it needs to be valid.
^\d{5}(?:,\d{5})+$
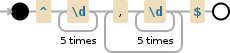
What if the user adds a space after the comma? Such as 12345, 12345
. This is perfectly valid, so let's make sure our validator allows that.
^\d{5}(?:,\s*\d{5})+$
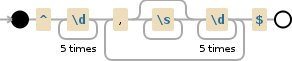
Oh, and zip codes can have an optional -1234
ending on them, too (known as ZIP+4. Maybe you want something like this
^\d{5}(?:-\d{4})?(?:,\s*\d{5}(?:-\d{4})?)+$

Now strings like this would be valid
- 12345
- 12345, 12345,12345
- 12345, 12345-9999, 12345
As a bonus, let's say 12345, 12345
is invalid because it has the same zip code twice. Here's how we'd fix that
(?:(\d{5}),?)(?!.*\1)
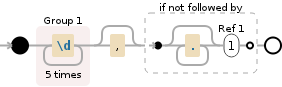
And here's the ZIP+4 version
(?:(\d{5}(?:-\d{4})?),?)(?!.*\1(?!-))
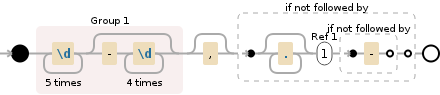
This one has a little added complexity because of possibility of (e.g.,) 12345, 12345-9999
. This is valid but because 12345
can appear more than once, it makes sure that a 5-digit zip code can't be invalidated by a unique 9-digit zip code.
Note these duplicate-checking regexps do not enforce the minimum of two unique zip codes. If you want to check for duplicates you'd need to combine the two.
var valid5DigitZipCodes = function(str) {
if (! /^\d{5}(?:,\s*\d{5})+$/.test(str)) {
alert("You need at least 2 zip codes");
return false;
}
else if (! /(?:(\d{5}),?)(?!.*\1)/.test(str)) {
alert("You entered a duplicate zip code");
return false;
}
return true;
};
And here's the ZIP+4 variant if you want to support that
var valid9DigitZipCodes = function(str) {
if (! /^\d{5}(?:-\d{4})?(?:,\s*\d{5}(?:-\d{4})?)+$/.test(str)) {
alert("You need at least 2 zip codes");
return false;
}
else if (! /(?:(\d{5}(?:-\d{4})?),?)(?!.*\1(?!-)).test(str) {
alert("You entered a duplicate zip code");
return false;
}
return true;
};