有两页。第一个是主页。这个执行一些伪计算。
根据这些计算,返回 Success.jsp 或 Failure.jsp。
这段代码将做你想要实现的......
尽管正如其他人指出的那样,最近有更高级的技术,但要跳舞,首先你必须知道动作......
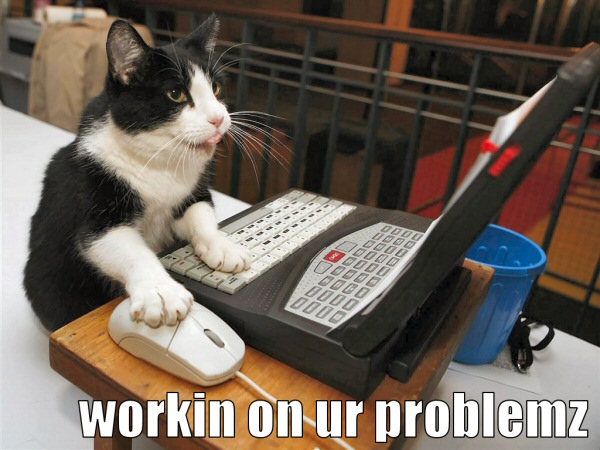
主要看这个 cObj.Print(request, response); 在第二个jsp页面中。
JSP 页面
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@ page import="java.util.*" %>
<%@ page import="rustler.Beans.Beany" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>JSP and JavaBean</title>
<%-- create an instance of Customer class --%>
<jsp:useBean id="cObj" scope="request" class="rustler.Beans.Beany">
<%-- Set the value of attribute such as CustID --%>
<jsp:setProperty name="cObj" property="*" />
</jsp:useBean>
</head>
<body>
<%
int x=cObj.setStoreCust();
if(x>=1)
{
%>
<jsp:forward page="Success.jsp" />
<%
}
else
{
%>
<jsp:forward page="Failure.jsp" />
<%
}
%>
</body>
</html>
JSP 页面
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@ page import="java.util.*" %>
<%@ page import="rustler.Beans.Beany" %>
<%@ page import="javax.servlet.http.HttpServletRequest" %>
<%@ page import="javax.servlet.http.HttpServletResponse" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Failure!</title>
<%-- create an instance of Customer class --%>
<jsp:useBean id="cObj" scope="request" class="rustler.Beans.Beany">
<%-- Set the value of attribute such as CustID --%>
<jsp:setProperty name="cObj" property="*" />
</jsp:useBean>
</head>
<body>
<%cObj.Print(request, response);%>
</body>
</html>
爪哇豆
package rustler.Beans;
import java.io.*;
import java.util.*;
import java.sql.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class Beany implements Serializable
{
public Beany()
{
}
/**
*
*/
private static final long serialVersionUID = 1L;
private String custID;
private String custName;
private int qty;
private float price;
private float total;
private int storeCust;
public String getCustID() {
return custID;
}
public void setJunk(String sStr)
{
//System.out.println("What a punk!");
custName = sStr;//"What a punk!";
}
public void Print(HttpServletRequest request,HttpServletResponse response)
{
try{
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.print("<p>haha</p>");
}catch(IOException e){
e.printStackTrace();
}
}
public String prntJunk()
{
//System.out.println("What a punk!");
return custName;//"What a punk!";
}
public void setCustID(String custID) {
this.custID = custID;
}
public String getCustName() {
return custName;
}
public void setCustName(String custName) {
this.custName = custName;
}
public int getQty() {
return qty;
}
public void setQty(int qty) {
this.qty = qty;
}
public float getPrice() {
return price;
}
public void setPrice(float price) {
this.price = price;
}
public float getTotal() {
return total;
}
public void setTotal(float total) {
this.total = total;
}
public int setStoreCust()
{
try{
Class.forName("com.mysql.jdbc.Driver");
Connection con=DriverManager.getConnection("jdbc:mysql://localhost:3306/usermaster","admin","password");
PreparedStatement pstmt=null;
String query=null;
query="insert into customer values(?,?,?,?,?)";
pstmt=con.prepareStatement(query);
pstmt.setString(1,custID);
pstmt.setString(2,custName);
pstmt.setInt(3,qty);
pstmt.setFloat(4,price);
pstmt.setFloat(5,total);
int i=pstmt.executeUpdate();
this.storeCust=i;
}
catch(Exception e)
{
}
return storeCust;
}
}